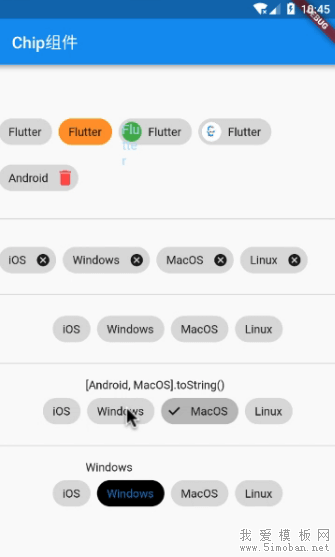
Chip组件非常强大,包括普通的Chip、ActionChiop、FilterChip、ChoiceChip,普通的Chip相当于tags标签,而且,这个标签还可以设置头像、图标,还有删除回调,而ActionChip则相当于可点击的Chip,FilterChip则是可以选择和取消选择的Chip,并且有选中状态,选中后前面会显示“√”,而ChoiceChip相当于单选的Chip,点击Chip,也有选中状态等,下面是详细的案例:
001 | class ChipDemo extends StatefulWidget { |
002 | ChipDemo({Key key}) : super (key: key); |
003 |
004 | @override |
005 | _ChipDemoState createState() => _ChipDemoState(); |
006 | } |
007 |
008 | class _ChipDemoState extends State<ChipDemo> { |
009 | List<String> _tags = [ 'Android' , 'IOS' , 'Windows' , 'macOS' , 'Linux' ]; |
010 | List<String> _selected = []; |
011 | String _choice = 'IOS' ; |
012 |
013 | @override |
014 | Widget build(BuildContext context) { |
015 | return Center( |
016 | child: Column( |
017 | mainAxisAlignment: MainAxisAlignment.center, |
018 | children: [ |
019 | Wrap( |
020 | spacing: 8.0, |
021 | runSpacing: 8.0, |
022 | children: [ |
023 | //普通圆角 |
024 | Chip( |
025 | label: Text( 'Flutter' ), |
026 | backgroundColor: Colors.orange, |
027 | ), |
028 |
029 | //带圆形头部的chip |
030 | Chip( |
031 | label: Text( 'Flutter' ), |
032 | avatar: CircleAvatar( |
033 | backgroundColor: Colors.green, |
034 | child: Text( 'F' ), |
035 | ), |
036 | ), |
037 |
038 | //带圆形头部并且是图片的chip |
039 | Chip( |
040 | label: Text( 'Flutter' ), |
041 | avatar: CircleAvatar( |
042 | backgroundImage: NetworkImage( 'http://www.5imoban.net/1.jpg' ), |
043 | ), |
044 | ), |
045 |
046 | //带删除按钮的chip |
047 | Chip( |
048 | label: Text( '张三' ), |
049 | //删除回调 |
050 | onDeleted: () {}, |
051 | deleteIcon: Icon(Icons. delete ), |
052 | deleteIconColor: Colors.red, |
053 | deleteButtonTooltipMessage: '删除这个标签' , |
054 | ) |
055 | ], |
056 | ), |
057 | //分割线 |
058 | Divider( |
059 | color: Colors.grey, |
060 | height: 36.0, |
061 | ), |
062 |
063 | //可删除的Chip案例,相当于tags标签 |
064 | Wrap( |
065 | spacing: 8.0, |
066 | runSpacing: 8.0, |
067 | children: _tags.map((tag) { |
068 | return Chip( |
069 | label: Text(tag), |
070 | onDeleted: () { |
071 | setState(() { |
072 | _tags.remove(tag); |
073 | }); |
074 | }, |
075 | ); |
076 | }).toList(), |
077 | ), |
078 | //分割线 |
079 | Divider( |
080 | color: Colors.grey, |
081 | height: 36.0, |
082 | ), |
083 |
084 | //可交互的Actionchip,相当于按钮 |
085 | Wrap( |
086 | spacing: 8.0, |
087 | runSpacing: 8.0, |
088 | children: _tags.map((tag) { |
089 | return ActionChip( |
090 | label: Text(tag), |
091 | onPressed: () { |
092 | print(tag); |
093 | }, |
094 | ); |
095 | }).toList(), |
096 | ), |
097 |
098 | //分割线 |
099 | Divider( |
100 | color: Colors.grey, |
101 | height: 36.0, |
102 | ), |
103 |
104 | //用来展示过滤的效果 |
105 | Container( |
106 | width: 300.0, |
107 | child: Text( '${_selected.toString()}' ), |
108 | ), |
109 | //FilterChip 过滤 |
110 | Wrap( |
111 | spacing: 8.0, |
112 | runSpacing: 8.0, |
113 | children: _tags.map((tag) { |
114 | return FilterChip( |
115 | label: Text(tag), |
116 | //当前的chip是否选中的效果 |
117 | selected: _selected.contains(tag), |
118 | onSelected: (value) { |
119 | setState(() { |
120 | if (_selected.contains(tag)) { |
121 | _selected.remove(tag); |
122 | } else { |
123 | _selected.add(tag); |
124 | } |
125 | }); |
126 | }, |
127 | ); |
128 | }).toList(), |
129 | ), |
130 |
131 | //分割线 |
132 | Divider( |
133 | color: Colors.grey, |
134 | height: 36.0, |
135 | ), |
136 |
137 | //用来展示选中的效果 |
138 | Container( |
139 | width: 300.0, |
140 | child: Text( '$_choice' ), |
141 | ), |
142 | //ChoiceChip 选择 |
143 | Wrap( |
144 | spacing: 8.0, |
145 | runSpacing: 8.0, |
146 | children: _tags.map((tag) { |
147 | return ChoiceChip( |
148 | label: Text(tag), |
149 | //当前的chip是否选中的效果 |
150 | selected: _choice == tag, |
151 | selectedColor: Colors.green, |
152 | onSelected: (value) { |
153 | setState(() { |
154 | _choice = tag; |
155 | }); |
156 | }, |
157 | ); |
158 | }).toList(), |
159 | ), |
160 | ], |
161 | ), |
162 | ); |
163 | } |
164 | } |