移动端触屏滑动的效果其实就是图片轮播,在PC的页面上很好实现,绑定click和mouseover等事件来完成。但是在移动设备上,要实现这种轮播的效果,就需要用到核心的touch事件。处理touch事件能跟踪到屏幕滑动的每根手指。
以下是四种touch事件:touchstart: //手指放到屏幕上时触发 touchmove: //手指在屏幕上滑动式触发 touchend: //手指离开屏幕时触发 touchcancel: //系统取消touch事件的时候触发,这个好像比较少用每个触摸事件被触发后,会生成一个event对象,event对象里额外包括以下三个触摸列表
touches: //当前屏幕上所有手指的列表 targetTouches: //当前dom元素上手指的列表,尽量使用这个代替touches changedTouches: //涉及当前事件的手指的列表,尽量使用这个代替touches这些列表里的每次触摸由touch对象组成,touch对象里包含着触摸信息,主要属性如下:
clientX / clientY: //触摸点相对浏览器窗口的位置 pageX / pageY: //触摸点相对于页面的位置 screenX / screenY: //触摸点相对于屏幕的位置 identifier: //touch对象的ID target: //当前的DOM元素注意:
手指在滑动整个屏幕时,会影响浏览器的行为,比如滚动和缩放。所以在调用touch事件时,要注意禁止缩放和滚动。
1.禁止缩放:通过meta元标签来设置
<meta name="viewport" content="target-densitydpi=320,width=640,user-scalable=no">2.禁止滚动:preventDefault是阻止默认行为,touch事件的默认行为就是滚动。
event.preventDefault();
案例:
下面给出一个案例,需在移动设备上才能看出效果。
1.定义touchstart的事件处理函数,并绑定事件:
if(!!self.touch) self.slider.addEventListener('touchstart',self.events,false); //定义touchstart的事件处理函数 start:function(event){ var touch = event.targetTouches[0]; //touches数组对象获得屏幕上所有的touch,取第一个touch startPos = {x:touch.pageX,y:touch.pageY,time:+new Date}; //取第一个touch的坐标值 isScrolling = 0; //这个参数判断是垂直滚动还是水平滚动 this.slider.addEventListener('touchmove',this,false); this.slider.addEventListener('touchend',this,false); },触发touchstart事件后,会产生一个event对象,event对象里包括触摸列表,获得屏幕上的第一个touch,并记下其pageX,pageY的坐标。定义一个变量标记滚动的方向。此时绑定touchmove,touchend事件。
2.定义手指在屏幕上移动的事件,定义touchmove函数。
//移动 move:function(event){ //当屏幕有多个touch或者页面被缩放过,就不执行move操作 if(event.targetTouches.length > 1 || event.scale && event.scale !== 1) return; var touch = event.targetTouches[0]; endPos = {x:touch.pageX - startPos.x,y:touch.pageY - startPos.y}; isScrolling = Math.abs(endPos.x) < Math.abs(endPos.y) ? 1:0; //isScrolling为1时,表示纵向滑动,0为横向滑动 if(isScrolling === 0){ event.preventDefault(); //阻止触摸事件的默认行为,即阻止滚屏 this.slider.className = 'cnt'; this.slider.style.left = -this.index*600 + endPos.x + 'px'; } },同样首先阻止页面的滚屏行为,touchmove触发后,会生成一个event对象,在event对象中获取touches触屏列表,取得第一个touch,并记下pageX,pageY的坐标,算出差值,得出手指滑动的偏移量,使当前DOM元素滑动。
3.定义手指从屏幕上拿起的事件,定义touchend函数。
//滑动释放 end:function(event){ var duration = +new Date - startPos.time; //滑动的持续时间 if(isScrolling === 0){ //当为水平滚动时 this.icon[this.index].className = ''; if(Number(duration) > 10){ //判断是左移还是右移,当偏移量大于10时执行 if(endPos.x > 10){ if(this.index !== 0) this.index -= 1; }else if(endPos.x < -10){ if(this.index !== this.icon.length-1) this.index += 1; } } this.icon[this.index].className = 'curr'; this.slider.className = 'cnt f-anim'; this.slider.style.left = -this.index*600 + 'px'; } //解绑事件 this.slider.removeEventListener('touchmove',this,false); this.slider.removeEventListener('touchend',this,false); },手指离开屏幕后,所执行的函数。这里先判断手指停留屏幕上的时间,如果时间太短,则不执行该函数。再判断手指是左滑动还是右滑动,分别执行不同的操作。最后很重要的一点是移除touchmove,touchend绑定事件。
实例演示:
<!doctype html> <html> <head> <meta charset='utf-8' /> <meta name="viewport" content="width=device-width,initial-scale=1.0,maximum-scale=1.0,user-scalable=no"/> <link rel="stylesheet" type="text/css" href="../css/reset-min.css"> <title></title> <style> .div{text-align: center;font-size:30px;} </style> </head> <body> <div class="div" style="width:100px;height:100px;line-height:100px;background-color:red;position:absolute;top:0;left:0;">1</div> <div class="div" style="width:100px;height:100px;line-height:100px;background-color:red;position:absolute;top:0;left:110px;">2</div> <div class="div" style="width:100px;height:100px;line-height:100px;background-color:red;position:absolute;top:0;left:220px;">3</div> <div class="div" style="width:100px;height:100px;line-height:100px;background-color:red;position:absolute;top:110px;left:0;">4</div> <div class="div" style="width:100px;height:100px;line-height:100px;background-color:red;position:absolute;top:110px;left:110px;">5</div> <div class="div" style="width:100px;height:100px;line-height:100px;background-color:red;position:absolute;top:110px;left:220px;">6</div> <script src="../js/jquery-3.0.0.min.js"></script> <script> function getcolor(){ var color_arr = ["0","1","2","3","4","5","6","7","8","9","a","b","c","d","e","f"]; function suiji(){ return Math.floor(Math.random()*16); } var colorSTR ="#"+color_arr[suiji()]+color_arr[suiji()]+color_arr[suiji()]+color_arr[suiji()]+color_arr[suiji()]+color_arr[suiji()]; return colorSTR; } var divd = $(".div"); divd.each(function(index){ $(this).on('touchstart', function(evt) { var e = event || evt; e.preventDefault();//阻止其他事件 //要做的事情 }).on('touchmove', function(evt) { var e = event || evt; e.preventDefault();//阻止其他事件 // 如果这个元素的位置内只有一个手指的话 //console.log(e.targetTouches) //console.log(event.targetTouches[0].clientX+"/"+event.targetTouches[0].clientY+"/"+event.targetTouches[0].pageX+"/"+event.targetTouches[0].pageY) if (e.targetTouches.length == 1) { var touch = e.targetTouches[0]; // 把元素放在手指所在的位置 $(this).css("left",(touch.pageX- parseInt($(this).width())/2 + 'px')); $(this).css("top",(touch.pageY- parseInt($(this).height())/2 + 'px')); $(this).css("background", getcolor()); } }).on('touchend', function(evt) { var e = event || evt; e.preventDefault();//阻止其他事件 $(this).css("background",getcolor()); }) }); </script> </body> </html>效果图(这些色块都可以随意拖动):
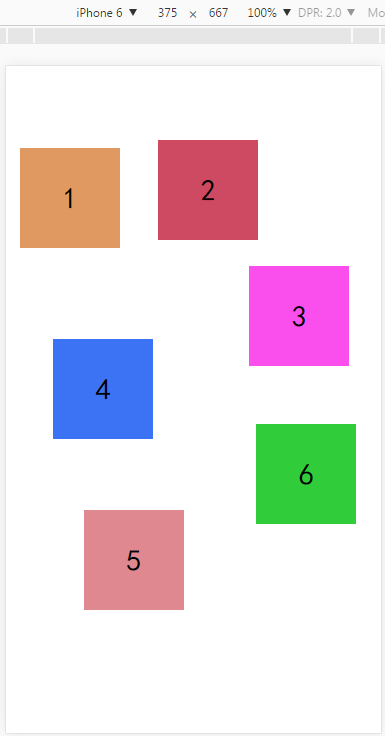